@RequestBody and @ResponseBody annotations are used to convert Body of HTTP request and response to Java class object. Both these annotations will use registered HTTP message converters in the process of converting/mapping HTTP request/response body with java objects.
What is HTTP message converter?
The Browser or Rest client cannot send Java Object (as it is) in the body of the HTTP request. and Server cannot compose the HTTP response body with Java Object (as it is). So both the HTTP Request and Response body cannot contain the as it is Java object.
These HTTP request and response object should contain data in either JSON, XML or some other data format. Therefore HTTP message converters are used to convert HTTP request body (either JSON or XML) to Java objects and java objects back to XML or JSON for composing HTTP response.
How does message converter work?
You must be confused about how java converts the message? Hoe spring framework identifies the content type to convert the Java Objects. This is achieved by checking the headers of the HTTP request. Let’s look at what are those headers in detailed.
Content Type
The header informs the server application about the Content Type of the body of the HTTP Request. Based on the content type specified in the Header information of the HTTP request, server applicati0on picks the correct message converter out the available message converter for converting the HTTP request body to the Java Object.
e.g:-
- If the Content-Type is application/json, then it will select a JSON to Java Object converter.
- If the Content-Type is application/xml, then it will select an XML to Java Object converter.
Accept
The header informs the server application about the Acceptable media type of the body of HTTP Response. Based on the value of Accept header, the server application picks the correct message converter out of the available message converters for converting the Java class (Object) to requested response type.
e.g:-
- If the Accept is application/json, then it will select a Java Object to JSON converter.
- If the Accept is application/xml, then it will select a Java Object to XML converter.
@RequestBody
In simple forms, we can say that @RequestBody annotation is used to map the HttpRequest body to a Java Object enabling automatic deserialization of the inbound HTTPRequest body to Java Object.
First, let us check the EmployeeController method,
@RestController
@RequestMapping("/api")
public class EmployeeController {
@Autowired
private EmployeeRepository employeeRepository;
@PostMapping(value = "/employee")
public Employee createEmployee(@RequestBody Employee employee)
{
return employeeRepository.save(employee);
}
}
Spring automatically deserialize the JSON into the Java type assuming the appropriate one is specified. By default, the Type (Object) we annotate with the @RequestBody must correspond to the JSON format when sent out from the client (Browser or Rest Client).
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue
private Long id;
private String name;
private String country;
private String email;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Now, let’s check out how the JSON data is passed from the client to the Controller method which will convert the HTTP message type into the appropriate Java Type.
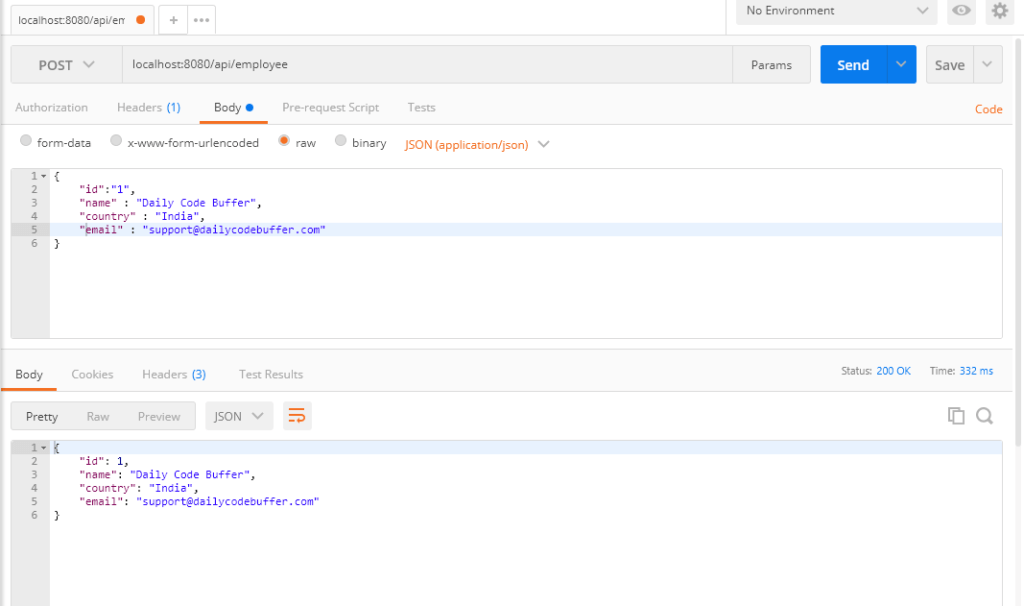
If we do not pass the data as specified as Java Type then it will not map to the particular attribute of Java Type. Considering in this example, a name attribute is defined in Java Type. Instead of name, If we pass ename, which is a different attribute name, it will not map to Java Object and its value will be null.
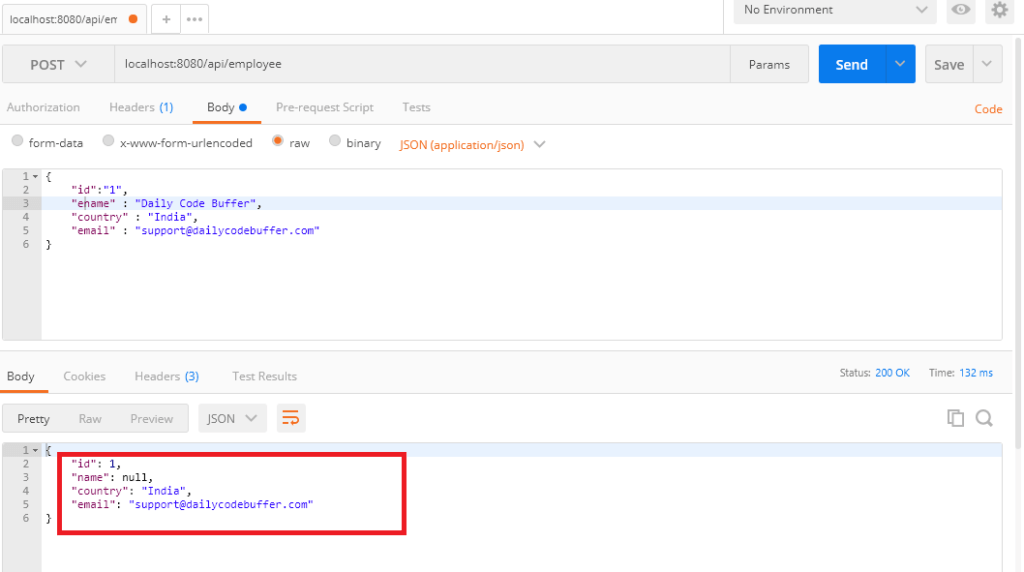
@ResponseBody
ResponseBody annotation is used to add a return value to the HTTP Response with the help of selected HTTP message converter. As seen earlier, the message converter is based on the value of Accept Header information of the HTTP header. @RestController is the most preferred way to achieve this functionality which was earlier provided by @ResponseBody. Under the hood in Spring framework, @Controller and @ResponseBody will behave the same as @RestController. Due to this, it avoids the need for prefixing the method with @ResponseBody.
package com.dailycodebuffer.examples.springboothttpconverter.Controller;
import com.dailycodebuffer.examples.springboothttpconverter.Entity.Employee;
import com.dailycodebuffer.examples.springboothttpconverter.Repository.EmployeeRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@Controller
@RequestMapping("/api")
public class EmployeeController {
@Autowired
private EmployeeRepository employeeRepository;
@ResponseBody
@GetMapping(value = "/employee/{id}")
public Employee getEmployeeById(@PathVariable Long id)
{
return employeeRepository.findById(id).get();
}
}
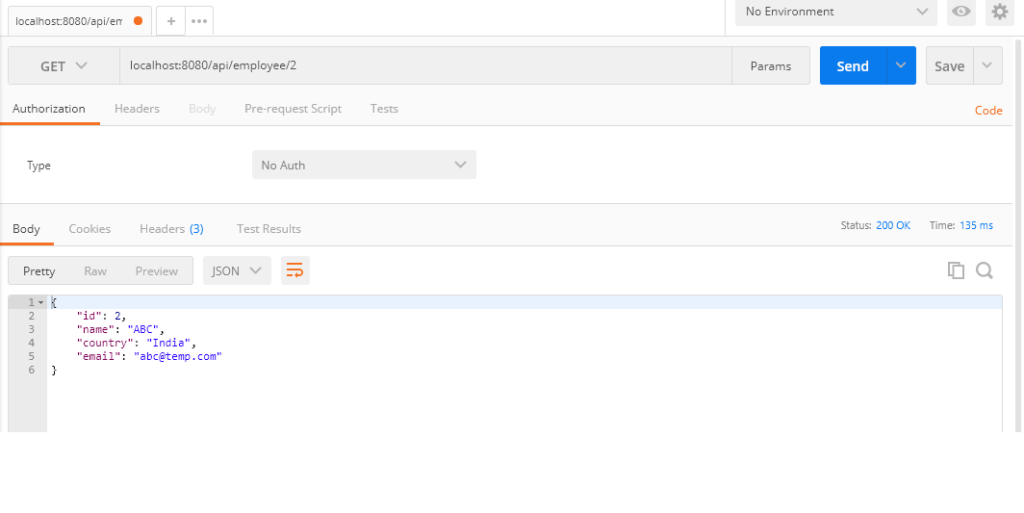
You can download or clone the Source code from Github
Click here to know more on Spring Framework.